If you’ve been using Ultimate Member and found yourself in need of a shortcode to showcase a user’s avatar image, you might have hit a roadblock. Ultimate Member does not include a default shortcode for displaying only a user’s avatar image. But don’t worry, we’ll walk you through the process of creating a shortcode that can dynamically display a user’s profile image that was uploaded using Ultimate Member. This customizable shortcode may be easily included into any page template to display a user’s avatar.
Shortcode for Displaying the Currently Logged-in User’s Image
Our first shortcode is designed to present the profile picture of the currently logged-in user. To boost SEO friendliness, we’ve added an alt property for the avatar image to improve SEO friendliness.
Usage: [um_profile_photo]
– Displays the currently logged-in user’s profile photo URL
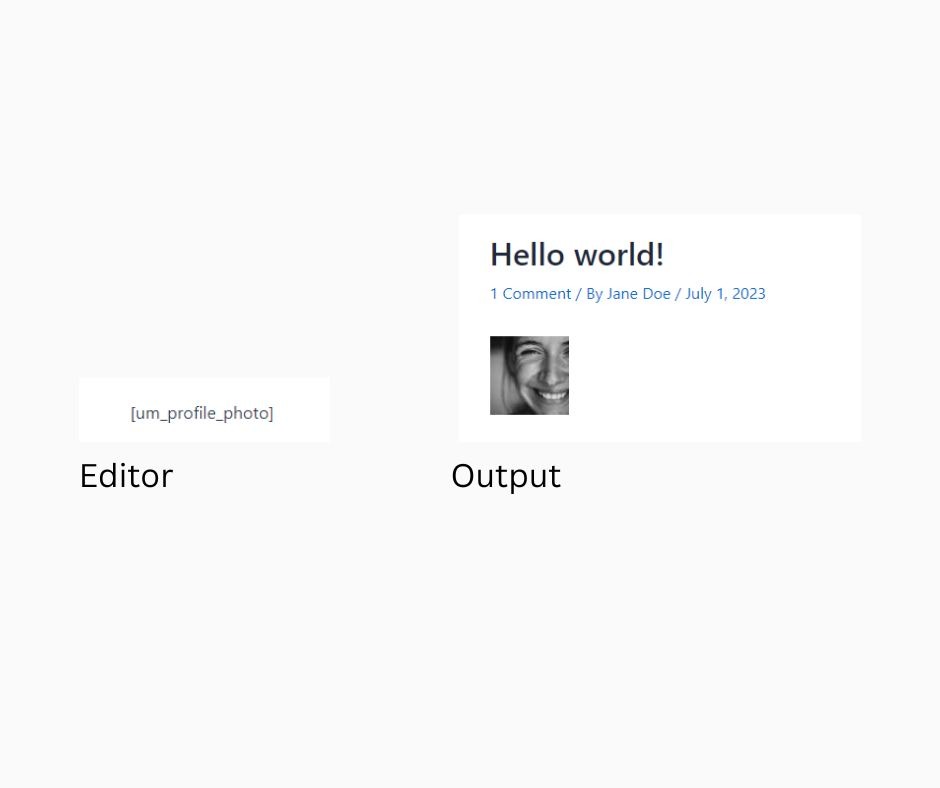
// Register the um_profile_photo shortcode
add_shortcode("um_profile_photo", "um_profile_photo_shortcode");
/**
* Callback function for um_profile_photo shortcode.
*/
function um_profile_photo_shortcode() {
// Get the user ID of the current profile
$user_id = get_current_user_id();
// Fetch user data for the current profile
um_fetch_user($user_id);
// Initialize the avatar URI with a default value
$avatar_uri = '';
// Get the URI of the current user's avatar (profile photo)
$avatar_uri = um_get_avatar_uri(um_profile('profile_photo'), 50);
$name = um_user( 'display_name' );
// If the avatar URI is empty, use the default UM avatar URI
if (empty($avatar_uri)) {
$url = um_get_default_avatar_uri();
} else {
$url = $avatar_uri;
}
um_reset_user();
// Output the profile photo URL
if (!empty($url)) {
echo '<img src="' . esc_url($url) . '" class="um-profile-img-shortcode" alt="' . esc_attr($name) . '"/>';
}
}
Shortcode for Displaying ANY Users Image
You can use this shortcode to display the profile photo or avatar of any Ultimate Member member. The key here is to know the user’s ID, which is required to use this shortcode successfully.
Usage: [um_profile_photo_id user_id="35"]
– Displays the profile photo URL of a specific user.
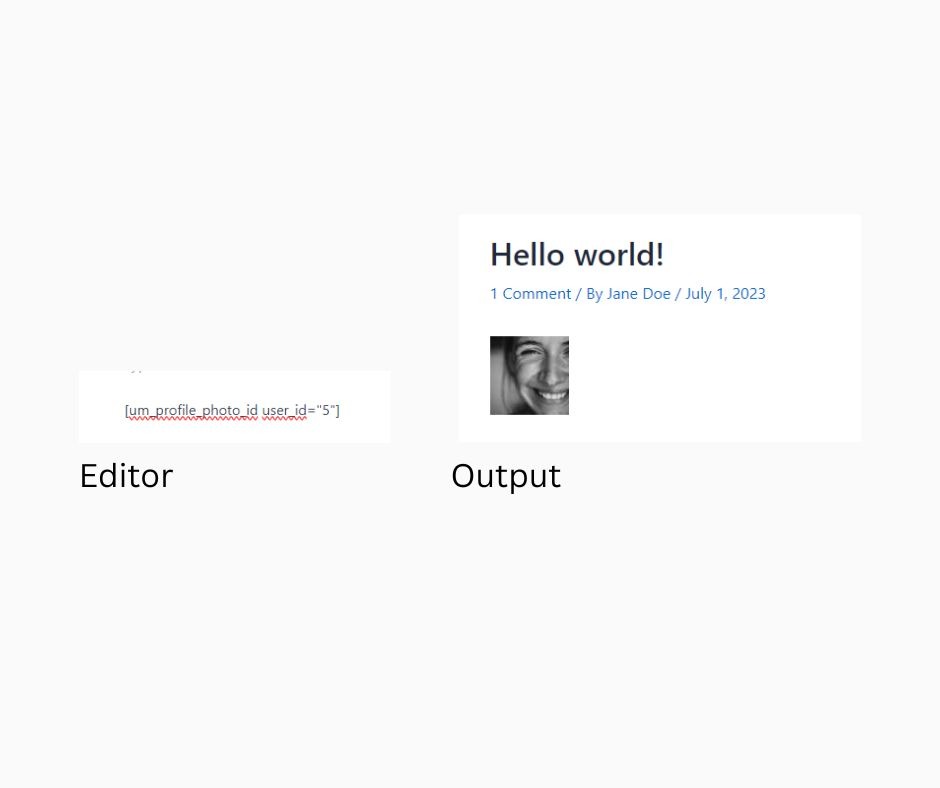
// Register the um_profile_photo shortcode
add_shortcode("um_profile_photo_id", "um_profile_photo_id_shortcode");
/**
* Callback function for um_profile_photo shortcode.
*
* @param array $atts Shortcode attributes.
*/
function um_profile_photo_id_shortcode($atts) {
// Parse shortcode attributes with default values
$atts = shortcode_atts(array(
'user_id' => get_current_user_id(), // Default to the current user's ID
), $atts);
// Get the user ID from the shortcode attribute
$user_id = intval($atts['user_id']);
// Fetch user data for the specified profile
um_fetch_user($user_id);
// Initialize the avatar URI with a default value
$avatar_uri = '';
// Get the URI of the user's avatar (profile photo)
$avatar_uri = um_get_avatar_uri(um_profile('profile_photo', $user_id), 50);
$name = um_user( 'display_name' );
// If the avatar URI is empty, use the default UM avatar URI
if (empty($avatar_uri)) {
$url = um_get_default_avatar_uri();
} else {
$url = $avatar_uri;
}
um_reset_user();
if (!empty($url)) {
echo '<img src="' . esc_url($url) . '" class="um-profile-img-shortcode" alt="' . esc_attr($name) . '"/>';
}
}
Where to Add this Code
You can either add it to your child theme’s functions.php file or opt for a user-friendly plugin such as Code Snippets to activate the custom code.
Trying to get a large profile photo image?
If you want to get an avatar or user image with larger resolution then increase the image size. The current code sets the image size to 50, meaning the width and height of the user image will be 50 pixels. If you prefer a larger version, simply set it to a value greater than 50.